CheckoutWithCard
The CheckoutWithCard element embeds a form on your app that accepts credit/debit card, Apple Pay, and Google Pay.
This component also handles:
- Apple Pay and Google Pay
- Bot and anti-fraud detection
- 3D Secure (if necessary)
- Buyer KYC (if necessary)
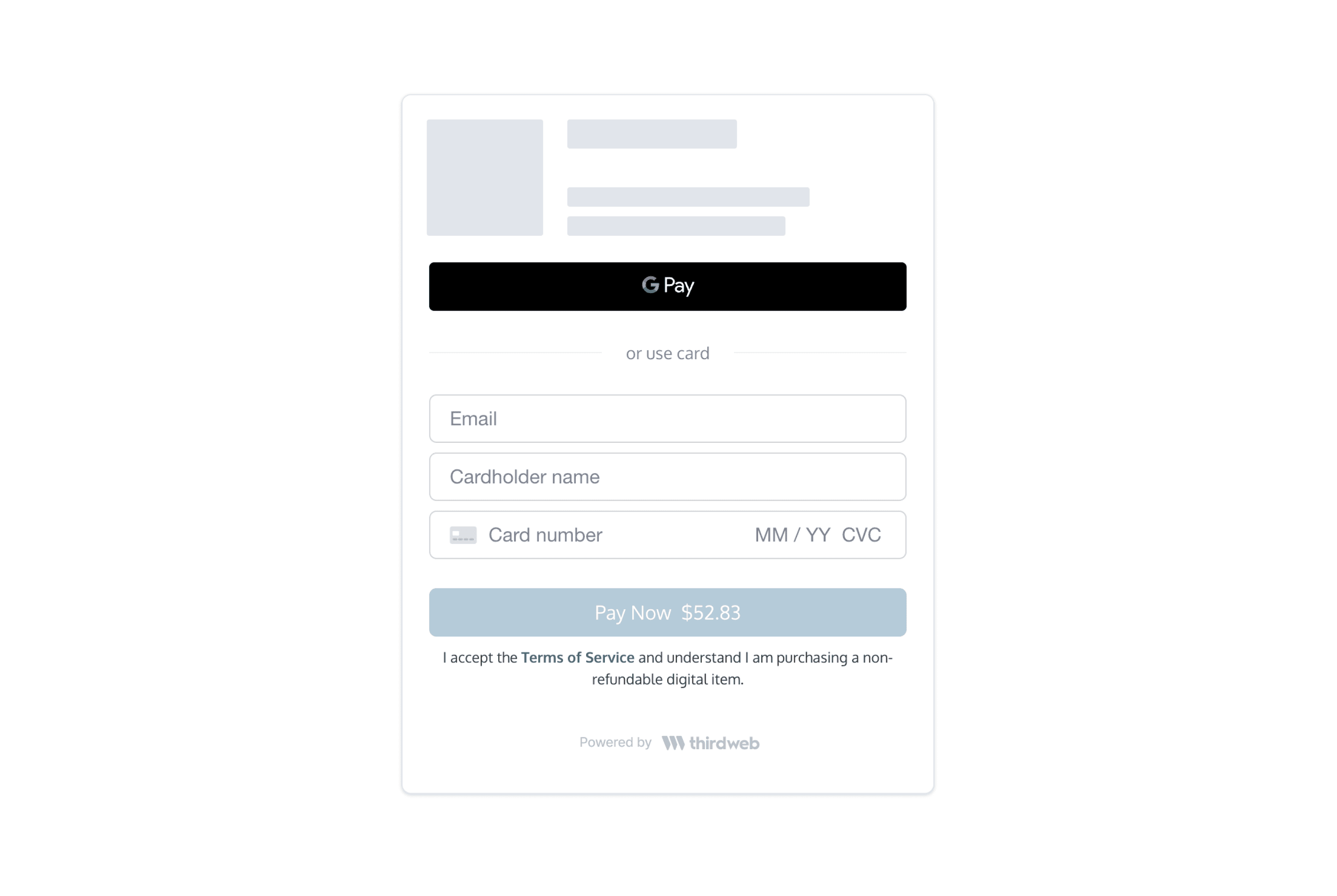
React Integration
Install the React SDK
Embed your component
On your frontend, render the
CheckoutWithCard
component with your configs.
Example
CheckoutWithCard
props
Javascript Integration
Install the Javascript SDK with your preferred package manager
Add your iframe
Call
createCheckoutWithCardElement
to insert the iframe on your page. Pass theconfigs
to this component.If you don't provide
elementOrId
, this call returns an iframe element for you to insert into your page.
Example code
Customization
The optional options
argument allows you to customize the component's styling. All customization fields are optional.
options
object
Example
Here's an example component with the following props:
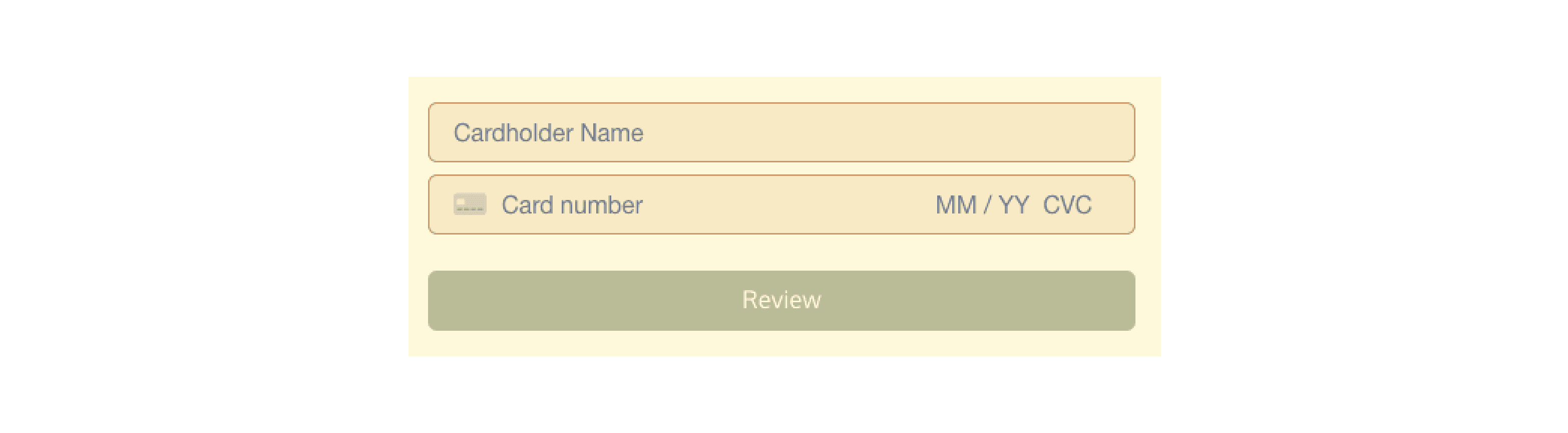